Core Java: An Integrated Approach, New: Includes All Versions upto Java 8
ISBN: 9789351199250
720 pages
For more information write to us at: acadmktg@wiley.com
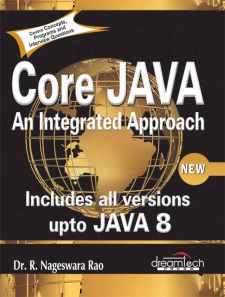
Description
Core Java – An Integrated Approach covers all core concepts in a methodical way. It helps you learn the concepts—from OOPS to abstract classes and interfaces; from software packaging to providing API documents; from error handling to converting fundamental data into object form; from collection framework to streams and creating client and server program to threads; from creating GUI applications to generics and communication with database. This book also covers the interview questions along with the subject matter to help students do well in interviews. The questions presented in this book have been collected from various interviews.
Chapter 1: All about Networks
- What Comprises the Internet?
Chapter 2: Introduction to Java
- Features of Java
- The Java Virtual Machine
- Parts of Java
Chapter 3: First Step towards Java Programming
- API Document
- Starting a Java program
- Formatting the Output
Chapter 4: Naming Conventions and Data Types
- Naming Conventions in Java
- Data Types in Java
- Literals
- Using Underscore in Numeric Literals
Chapter 5: Operators in Java
- Operators
- Priority of Operators
Chapter 6: Control Statements in Java
- if…else Statement
- do…while Loop
- while Loop
- for Loop
- switch Statement
- break Statement
- continue Statement
- return Statement
Chapter 7: Input and Output
- Accepting Input from the Keyboard
- Reading Input with java.util.Scanner Class
- Displaying Output with System.out.printf()
- Displaying Formatted Output with String.format()
Chapter 8: Arrays
- Types of Arrays
- Three dimensional arrays (3D array)
- arrayname.length
- Command Line Arguments
Chapter 9: Strings
- Creating Strings
- String Class Methods
- String Comparison
- Immutability of Strings
Chapter 10: StringBuffer and StringBuilder
- Creating StringBuffer Objects
- StringBuffer Class Methods
- StringBuilder Class
- StringBuilder Class Methods
Chapter 11: Introduction to OOPs
- Problems in Procedure Oriented Approach
- Features of Object Oriented Programming System (OOPS)
- Polymorphism
Chapter 12: Classes and Objects
- Object Creation
- Initializing the Instance Variables
- Access Specifiers
- Constructors
Chapter 13: Methods in Java
- Method Header or Method Prototype
- Method Body
- Understanding Methods
- Static Methods
- Static Block
- The keyword ‘this’
- Instance Methods
- Passing Primitive Data Types to Methods
- Passing Objects to Methods
- Passing Arrays to Methods
- Recursion
- Factory Methods
Chapter 14: Relationship Between Objects
- Relating Objects using References
- Inner Class
Chapter 15: Inheritance
- Inheritance
- The Keyword ‘super’
- The Protected Specifier
- Types of Inheritance
Chapter 16: Polymorphism
- Polymorphism with Variables
- Polymorphism using Methods
- Polymorphism with Static Methods
- Polymorphism with Private Methods
- Polymorphism with Final Methods
- final Class
Chapter 17: Type Casting
- Types of Data Types
- Casting Primitive Data Types
- Casting Referenced Data Types
- The Object Class
Chapter 18: Abstract Classes
- Abstract Method and Abstract Class
Chapter 19: Interfaces
- Interface
- Multiple Inheritance using Interfaces
- Callbacks using Interfaces
Chapter 20: Packages
- Package
- Different Types of Packages
- The JAR Files
- Interfaces in a Package
- Creating Sub Package in a Package
- Access Specifiers in Java
- Creating API Document
Chapter 21: Exception Handling
- Errors in a Java Program
- Exceptions
- A different Try Statement
- throws Clause
- throw Clause
- Types of Exceptions
- Re-throwing an Exception
Chapter 22: Wrapper Classes
- Wrapper Classes
- Number Class
- Character Class
- Byte Class
- Short Class
- Integer Class
- Long Class
- Float Class
- Double Class
- Boolean Class
- Math Class
Chapter 23: The Collection Framework
- Using an Array to Store a Group of Objects
- Collection Objects
- Maps
- Retrieving Elements from Collections
- HashSet Class
- LinkedHashSet Class
- Stack Class
- LinkedList Class
- ArrayList Class
- ArrayList Class Methods
- Vector Class
- Queue Interface
- HashMap Class
- Hashtable Class
- Arrays Class
- Using Comparator to Sort an Array
- StringTokenizer Class
- Calendar Class
- Date Class
Chapter 24: Streams and Files
- Stream
- Creating a file using FileOutputStream
- Reading Data from a File using FileInputStream
- Creating a File using FileWriter
- Reading a File using FileReader
- Storing strings into a file using FileWriter
- Reading strings from a file using FileReader
- Zipping and Unzipping Files
- Serialization of Objects
- Counting Number of Characters in a File
- File Copy
- File Class
Chapter 25: Networking in Java
- TCP/IP Protocol
- User Datagram Protocol (UDP)
- Sockets
- Knowing IP Address
- URL
- URLConnection Class
- Creating a Server That Sends Data
- Creating a Client That Receives Data
- Two-way Communication between Server and Client
- Retrieving a file at server
Chapter 26: Threads
- Single Tasking
- Multi Tasking
- Uses of Threads
- Creating a Thread and Running it
- Terminating the Thread
- Single Tasking Using a Thread
- Multi Tasking Using Threads
- Multiple Threads Acting on Single Object
- Thread Class Methods
- Deadlock of Threads
- Thread Communication
- Thread Priorities
- Thread Group
- Daemon Threads
- Applications of Threads
- Thread Pools
- Types of Thread Pools
- Thread Life Cycle
Chapter 27: Graphics Programming using AWT
- AWT
- Components
- Window and Frame
- Creating a Frame
- Event Delegation Model
- Closing the Frame
- Uses of a Frame
- Drawing in the Frame
- Filling with Colors
- Displaying Dots
- Displaying text in the frame
- Knowing the Available Fonts
- Displaying Images in the Frame
- Component Class Methods
- Push Buttons
- Listeners and Listener Methods
- Check Boxes
- Radio Button
- TextField
- TextArea
- Label
- Choice Class
- List Class
- Scrollbar Class
- Knowing the Keys on Keyboard
- Working with Several Frames
Chapter 28: Graphics Programming using Swing
- Java Foundation Classes (JFC)
- javax.swing and MVC
- Window Panes
- Important Classes of javax.swing
- Creating a Frame in Swing
- Displaying Text in Frame
- JComponent Class Methods
- Creating a Push Button with All Features
- Displaying Image in Swing
- Creating Components in Swing
- Setting the Look and Feel of Components
- JTable Class
- JTabbedPane Class
- JSplitPane Class
- JTree Class
- JComboBox Class
- JList Class
- JMenu Class
- JToggleButton Class
- JProgressBar Class
- JToolBar Class
- JColorChooser Class
- Handling Keyboard Events
- Handling Mouse Events
Chapter 29: Graphics Programming – Layout Managers
- FlowLayout
- BorderLayout
- CardLayout
- Using a Layout Inside Another Layout
- GridLayout
- GridBagLayout
- BoxLayout
- Box Class
Chapter 30: Applets
- Creating an Applet
- Uses of Applets
- A Simple Applet
- An Applet with Swing Components
- Animation in Applets
- A Simple Game with an Applet
- Applet Parameters
Chapter 31: Generic Types
- Generic Class
- Generic Method
- Generic Interface
Chapter 32: Java Database Connectivity
- Database Servers
- Database Clients
- JDBC (Java Database Connectivity)
- Working with Oracle Database
- Working with MySQL Database
- Stages in a JDBC Program
- Registering the Driver
- Connecting to a Database
- Preparing SQL Statements
- Using jdbc-odbc Bridge Driver to Connect to Oracle Database
- Retrieving Data from MySQL Database
- Retrieving Data from MS Access Database
- Improving the Performance of a JDBC Program
- Affect of Driver
- Affect of setFetchSize()
- Affect of PreparedStatement
- Stored Procedures and CallableStatement
- Types of Result Sets
- Storing Images into Database
- Retrieving Images from Database
- Storing a file into database
- Retrieving a File from the Database
- ResultSetMetaData
- DatabaseMetaData
- Types of JDBC Drivers
Chapter 33: Enumerations and Annotations
- Enumerations
Chapter 34: Lambda Expressions
- Lambda Expressions and Functional interfaces
- Points regarding construction of the lambda expressions
- Functional interface
- Accessing variables using Lambda expressions
- The need of lambda expressions
- Passing lambda expressions as arguments to a method
- Passing lambda expressions to objects
- Default Methods
- Predicates
- Joining the predicates
- Functions
- Double colon operator (::)
Chapter 35: Streams API
- Creating streams
- Operations on streams
- filter() method
- map() method
- sorted() method
- forEach() method
- count() method
- collect() method
Chapter 36: Joda-Time
- Joda-Time
- Important classes in java.time package
- Method naming conventions
Index I - Question Index
Index II - Program Index